Laravel Carbon Check Current Date Between Two Dates Example
How to check if a date is between two dates using Carbon in Laravel, with a simple example provided. This guide is applicable to Laravel 6, 7, 8, 9, and 10 applications.
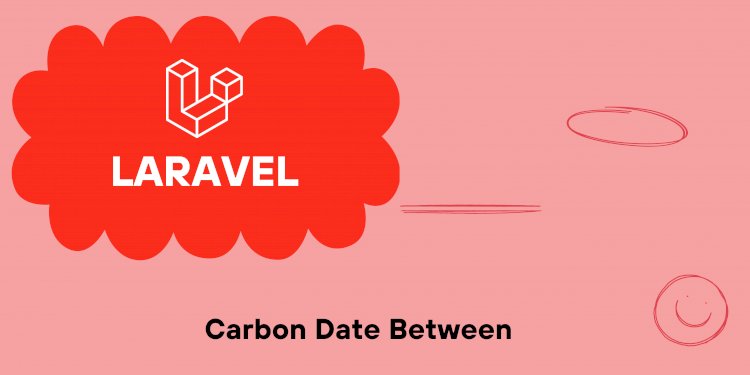
This tutorial will provide an example of how to use Laravel Carbon to check if a date falls between two given dates. You will learn how to check if a date is between two dates using Carbon in Laravel, with a simple example provided. This guide is applicable to Laravel 6, 7, 8, 9, and 10 applications.
By following the steps outlined in this tutorial, you will be able to check if the current date falls between two dates using Carbon in Laravel. The example provided will give you a better understanding of how to use Carbon to check if a date falls within a given range.
use Carbon\Carbon;
// Set the start and end dates as Carbon objects
$start_date = Carbon::parse('2022-01-01');
$end_date = Carbon::parse('2022-12-31');
// Get the current date as a Carbon object
$current_date = Carbon::now();
// Check if the current date is between the start and end dates
if ($current_date->between($start_date, $end_date)) {
echo 'The current date is between the start and end dates.';
} else {
echo 'The current date is not between the start and end dates.';
}
In this code, we first import the Carbon
class from the Carbon\Carbon
namespace. We then define the start and end dates as strings, and use the Carbon::now()
method to get the current date as a Carbon
object. Finally, we use the between()
method on the current date to check if it falls between the start and end dates. If it does, we output a message saying so. Otherwise, we output a message saying it does not.
This code should work in any version of Laravel that supports Carbon.